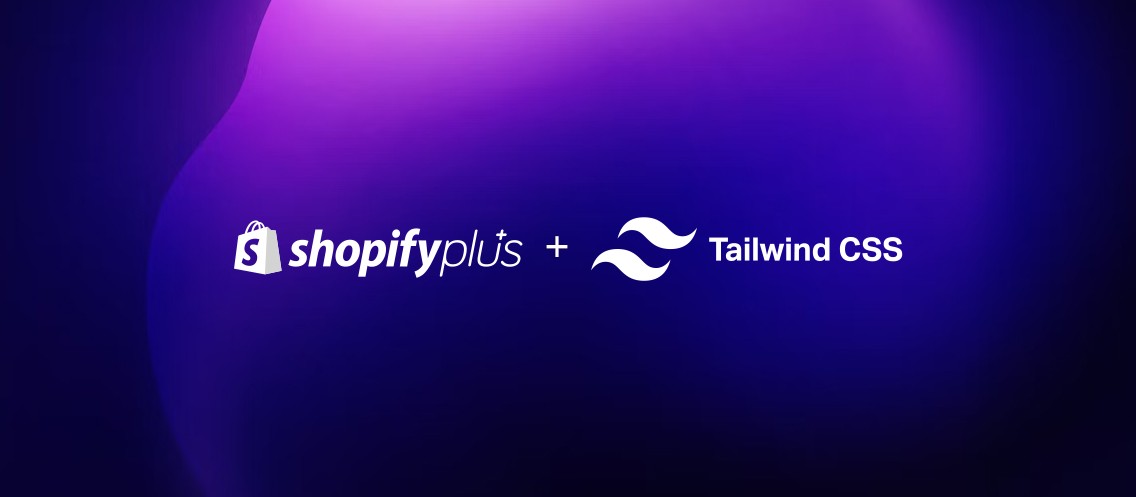
Shopify Theme Development for Store 2.0 using TailwindCSS
As a leading eCommerce web design agency, we specialize in customizing Shopify themes for merchants. Our services range from minor adjustments to comprehensive redesigns. As a Shopify expert partner, we are dedicated to providing theme customization services for your online store to enhance its overall look and feel.
In this tutorial we show you how to Customize an existing Shopify Theme Store 2.0 using TailwindCSS.
What you'll learn:
- Install Shopify CLI
- Download the merchant's theme code
- Configured your local development environment
- Obtained a copy of the merchant's theme
- Implemented modifications and previewed them
- Implemented and deployed the modifications live
- Install Tailwind CSS with Shopify Theme CLI Store 2.0 and Webpack
Requirements
The URL of the online store you wish to modify, such as johns-apparel.myshopify.com.
Step 1: Install Shopify CLI
Before proceeding, ensure that you have Shopify CLI installed. Shopify CLI is a command-line tool that aids in building Shopify themes, it allows for the preview, testing and sharing of changes to themes during local development. Below are the instructions for installation on macOS, Windows, or Linux.
macOS (Homebrew)
> brew tap shopify/shopify
> brew install shopify-cli
Step 2: Download the merchant's theme code
Run the following command to retrieve a list of all of the themes in the store. You can optionally specify the local path where the theme should be stored using the --path flag.
> shopify theme pull --store johns-apparel.myshopify.com
example:
> shopify theme pull --store {store-name}
Choose a theme from the provided list, its files will be downloaded to the current directory or the specified directory.
Tip
You will be prompted to log in to Shopify upon executing the pull command, if you haven't done so previously. Ensure that you use the account which has been granted access to the store. If you are logged in with an account that lacks the necessary permissions, you can log out by running the command 'shopify auth logout'.
Step 3: Customization the theme
Once you have obtained a copy of the merchant's theme, you can make the required modifications to the theme code. For instance, you can make changes to the theme's CSS to alter its visual design.
If you haven't already, we suggest that you initialize your git repository and commit your initial changes by running the following commands:
> git init
> git add .
> git commit -m "initial commit"
Step 4: Preview your changes
Once you have made modifications to the theme, you can run the command 'shopify theme dev' to preview the theme in a browser. Shopify CLI will upload the theme as a development theme to the store that you are connected to.
This command will return a URL that will automatically reflect the changes made in the CSS and sections in real-time, utilizing the store's data. It should be noted that this preview feature is only compatible with Google Chrome.
> shopify theme dev
Using your Google Chrome browser, navigate to your local environment using http://127.0.0.1:9292 to open the theme preview.
Upon running the command 'shopify auth logout', the development theme will be deleted. In case you wish to share your progress with the merchant, proceed to the following step.
Step 5: Share your changes
To share the modifications you made with the merchant, you will need to upload the changes to their store. You will be prompted to select the theme you wish to update. This command will provide you with a link to the theme editor in the Shopify admin, as well as a preview link, which can be shared with the merchant.
> shopify theme push
If you do not wish to update an existing theme in the store with your changes, you can upload your theme to the theme library as a new and unpublished theme by using the --unpublished flag.
Step 6: Publish the updated theme
Once the merchant has reviewed and approved the modifications, you can publish the theme to make it live in the merchant's store. Prior to publishing the theme, ensure that your changes have been uploaded to the store and that you have committed your changes using git.
> shopify theme publish
Select the theme that you want to publish from the list and select Yes to confirm that you want to publish the specified theme.
Step 7: Install Tailwind CSS with Shopify Theme CLI Store 2.0 and Webpack
We will be using Laravel Mix for watching our compile files. Laravel Mix is a tool used for compiling and bundling JavaScript and CSS assets in a Laravel application. It is an abstraction on top of popular front-end build tools such as webpack, which can be difficult to configure for beginners. Laravel Mix provides a simple, fluent API for defining webpack build steps for your application using several common CSS and JavaScript pre-processors.
With Laravel Mix, you can write and manage your application's front-end assets using a single config file, webpack.mix.js, which is located in the root of your project directory. This config file defines all the necessary settings for compiling and bundling your application's assets, such as which pre-processors to use, where to find your source files, and where to output the final bundled files.
Initiate your NPM package.json by running the following command:
> npm init -y
Install Laravel Mix by running this command:
> npm install laravel-mix --save-dev
Create the following directories and files in the root of your project:
> mkdir src
> mkdir src/js
> mkdir src/scss
> touch src/js/app.js
> touch src/scss/app.scss
Create the webpack.mix.js file and add the following code:
> touch webpack.mix.js
add:
// webpack.mix.js
let mix = require('laravel-mix');
const tailwindcss = require('tailwindcss')
mix.js('src/js/app.js', 'assets')
.sass('src/scss/app.scss', 'assets');
Run a test:
> vim src/scss/app.scss
add:
body {
background-color: red;
}
Compile your code:
We have now prepared all necessary assets, Mix offers a command-line interface named 'mix' which initiates the corresponding webpack build. Let's execute it now.
> npx mix
You should now see a file in the assets directory called app.css with the following output
body {
background-color: red;
}
Install TailwindCSS
Now we will install Tailwind CSS. Tailwind CSS is a utility-first CSS framework. It provides a set of pre-defined CSS classes that you can use to apply common styling to your HTML elements, such as text colors, spacing, and layout. By using these classes, you can quickly apply styles to your elements without having to write custom CSS.
The main idea behind Tailwind CSS is to give developers a set of low-level utility classes that they can use to build their own custom design. Instead of providing a set of pre-designed UI components, Tailwind CSS focuses on providing a large set of small, composable CSS classes that can be combined in various ways to create a wide variety of design patterns.
> npm install -D tailwindcss autoprefixer postcss
> npx tailwindcss init
> touch postcss.config.js
Add the following code to your tailwind.config.js file that generate:
> vim tailwind.config.js
add:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
'./config/*.json',
'./layout/*.liquid',
'./assets/*.liquid',
'./sections/*.liquid',
'./snippets/*.liquid',
'./templates/*.liquid',
'./templates/*.json',
'./templates/customers/*.liquid',
'./locales/*.json',
],
theme: {
extend: {},
},
plugins: [],
}
In the above example you are watching for changes to the following directories and extension files.
Now include the include tailwind css libraries in your app.scss file, make sure that it is NOT the the app.css file store in the assets folder:
> vim src/scss/app.scss
add:
@tailwind base;
@tailwind components;
@tailwind utilities;
Update your webpack.mix.js file to include the following options code:
> vim webpack.mix.js
add:
// webpack.mix.js
let mix = require('laravel-mix');
const tailwindcss = require('tailwindcss')
mix.js('src/js/app.js', 'assets')
.sass('src/scss/app.scss', 'assets')
.options({
processCssUrls: false,
postCss: [ tailwindcss('tailwind.config.js')],
});
Run the Laravel Mix command to compile the code:
> npx mix
Final step is to include the app.css file that gets generated by Laravel Mix in your theme.liquid file:
> vim theme.liquid
add:
{{ 'app.css' | asset_url | stylesheet_tag }}
...
</head>
Now to watch the Laravel Mix files run the following command:
> npx mix watch
and to sync preview your changes run:
> shopify theme dev
You can now add any Tailwind CSS class to any of your div for example: bg-red-500
<div class="bg-red-500"></div>
Make sure to commit your changes to your git repository and to include the node_modules directory on your .gitignore file:
> touch .gitignore
add:
node_modules
Experience the benefits of working with us
Fill out the form below and we will contact you shortly.